Greetings fellow developers! In the era of ever-increasing cyber threats, ensuring the security of our web applications has never been more critical. With PHP being one of the most widely used server-side scripting languages, it’s essential for us to understand and apply best practices to maintain a secure environment. In this post, we’ll delve into the top five security practices every PHP developer should follow.
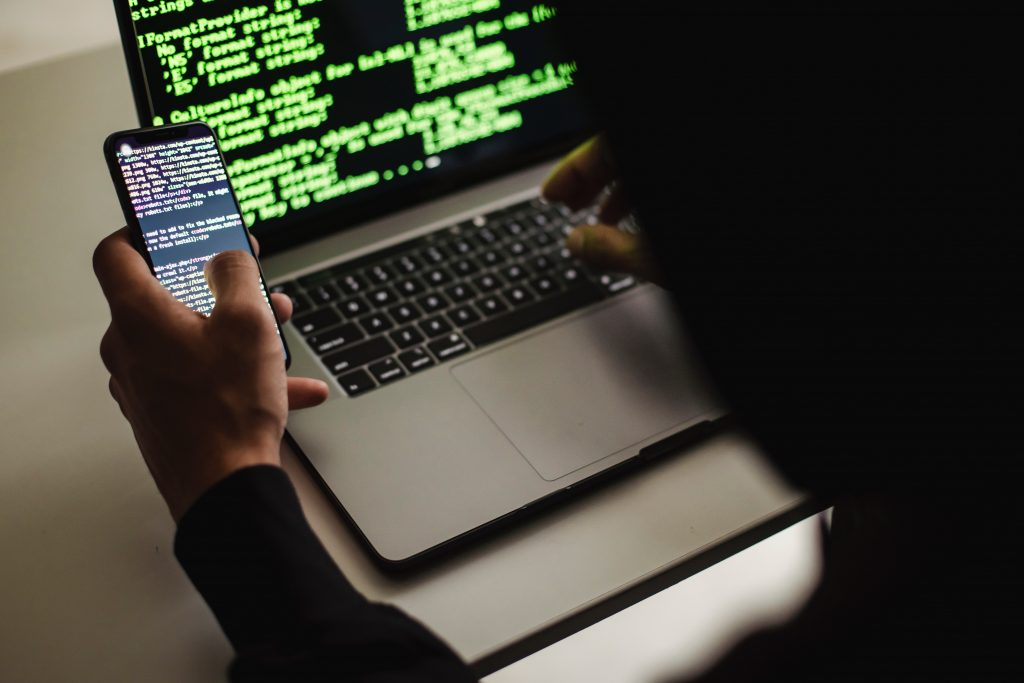
1. Sanitize User Input
Do: Always sanitize and validate user inputs. As a rule of thumb, treat all user inputs as potentially malicious. Make use of PHP’s filter functions, such as filter_input
and filter_var
, to sanitize input data.
$username = filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING);
$email = filter_input(INPUT_POST, 'email', FILTER_SANITIZE_EMAIL);
Don't: Never use user input directly without sanitizing or validating it first. This can open up your application to several vulnerabilities, including SQL injection and cross-site scripting (XSS).
2. Use Prepared Statements for Database Queries
Do: Always use prepared statements or parameterized queries for database operations. This prevents SQL injection attacks, where malicious SQL code is inserted into a query. Both PDO and MySQLi support prepared statements.
// PDO
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email');
$stmt->execute(['email' => $email]);
$user = $stmt->fetch();
// MySQLi
$stmt = $mysqli->prepare('SELECT * FROM users WHERE email = ?');
$stmt->execute([$email]);
$user = $stmt->get_result()->fetch_assoc();
Don’t: Avoid using old, unsafe functions like mysql_query
. Also, refrain from directly inserting user input into your SQL queries.
3. Enforce Strong Password Policies
Do: Use PHP’s built-in functions for handling password hashing and verification, such as password_hash
and password_verify
. Never store plain-text passwords; always store hashed versions.
// Hashing a password
$hashedPassword = password_hash($password, PASSWORD_DEFAULT);
// Verifying a password
if (password_verify($inputPassword, $hashedPassword)) {
// Password is valid
}
Don’t: Don’t use weak or outdated hashing algorithms like MD5 or SHA1.
4. Protect Against Cross-Site Request Forgery (CSRF)
Do: Implement CSRF tokens in your forms to protect against CSRF attacks. A CSRF token is a random value associated with a user’s session, which is expected with each subsequent request.
session_start();
$_SESSION['token'] = bin2hex(random_bytes(32));
// In the form
echo '<input type="hidden" name="token" value="' . $_SESSION['token'] . '">';
Don’t: Don’t leave your forms without CSRF protection, especially if they trigger state changes.
5. Use Secure Cookies and Sessions
Do: Set the HttpOnly
, Secure
, and SameSite
attributes for your cookies. HttpOnly
prevents scripts from accessing the cookie, Secure
ensures the cookie is only sent over HTTPS, and SameSite
restricts the cookie to first-party contexts.
setcookie('key', 'value', [
'expires' => time() + 3600,
'path' => '/',
'domain' => 'example.com',
'secure' => true,
'httponly' => true,
'samesite' => 'Strict',
]);
Don’t: Don’t ignore the security attributes when setting cookies.
In conclusion, web security is a critical aspect of PHP development that shouldn’t be taken lightly. By following these practices, you can help protect your web applications from common threats and ensure a safer user experience. Always remember: security isn’t a one-time task but an ongoing commitment.
+ There are no comments
Add yours